工具调用
工具调用(也称为函数调用)是一种在 AI 应用中常见的模式,允许模型与一组 API(或工具)交互,从而增强其能力。
工具主要用于
-
信息检索。此类工具可用于从外部来源检索信息,例如数据库、Web 服务、文件系统或 Web 搜索引擎。其目标是增强模型的知识,使其能够回答原本无法回答的问题。因此,它们可用于检索增强生成(RAG)场景。例如,工具可用于检索给定位置的当前天气、检索最新新闻文章或查询数据库以获取特定记录。
-
执行操作。此类工具可用于在软件系统中执行操作,例如发送电子邮件、在数据库中创建新记录、提交表单或触发工作流。其目标是自动化原本需要人工干预或显式编程的任务。例如,工具可用于为与聊天机器人交互的客户预订航班、填写网页上的表单,或在代码生成场景中根据自动化测试 (TDD) 实现 Java 类。
尽管我们通常将工具调用称为模型的能力,但实际上是由客户端应用提供工具调用逻辑。模型只能请求工具调用并提供输入参数,而应用负责根据输入参数执行工具调用并返回结果。模型永远无法访问作为工具提供的任何 API,这是一个重要的安全考虑因素。
Spring AI 提供了方便的 API 来定义工具、解析来自模型的工具调用请求并执行工具调用。以下部分概述了 Spring AI 中的工具调用能力。
查看Chat 模型对比,以查看哪些 AI 模型支持工具调用。 |
快速入门
让我们看看如何在 Spring AI 中开始使用工具调用。我们将实现两个简单工具:一个用于信息检索,一个用于执行操作。信息检索工具将用于获取用户时区的当前日期和时间。执行操作工具将用于为指定时间设置闹钟。
信息检索
AI 模型无法访问实时信息。任何假设了解当前日期或天气预报等信息的问题,模型都无法回答。但是,我们可以提供一个能够检索这些信息的工具,并在需要访问实时信息时让模型调用此工具。
让我们在 DateTimeTools
类中实现一个获取用户时区当前日期和时间的工具。该工具将不接受任何参数。Spring Framework 中的 LocaleContextHolder
可以提供用户时区。该工具将被定义为一个使用 @Tool
注解的方法。为了帮助模型理解何时以及如何调用此工具,我们将提供该工具功能的详细描述。
import java.time.LocalDateTime;
import org.springframework.ai.tool.annotation.Tool;
import org.springframework.context.i18n.LocaleContextHolder;
class DateTimeTools {
@Tool(description = "Get the current date and time in the user's timezone")
String getCurrentDateTime() {
return LocalDateTime.now().atZone(LocaleContextHolder.getTimeZone().toZoneId()).toString();
}
}
接下来,让我们使该工具对模型可用。在此示例中,我们将使用 ChatClient
与模型交互。我们将通过 tools()
方法传递 DateTimeTools
的实例,从而向模型提供该工具。当模型需要知道当前日期和时间时,它将请求调用该工具。在内部,ChatClient
将调用该工具并将结果返回给模型,模型随后将使用工具调用结果生成对原始问题的最终响应。
ChatModel chatModel = ...
String response = ChatClient.create(chatModel)
.prompt("What day is tomorrow?")
.tools(new DateTimeTools())
.call()
.content();
System.out.println(response);
输出将类似于
Tomorrow is 2015-10-21.
您可以再次尝试询问相同的问题。这次,不要将该工具提供给模型。输出将类似于
I am an AI and do not have access to real-time information. Please provide the current date so I can accurately determine what day tomorrow will be.
没有该工具,模型不知道如何回答该问题,因为它没有确定当前日期和时间的能力。
执行操作
AI 模型可用于生成实现某些目标的计划。例如,模型可以生成预订前往丹麦旅行的计划。但是,模型没有执行该计划的能力。这就是工具的作用所在:它们可用于执行模型生成的计划。
在上一个示例中,我们使用了工具来确定当前日期和时间。在此示例中,我们将定义第二个工具,用于在特定时间设置闹钟。目标是设置一个从现在起 10 分钟后的闹钟,因此我们需要将这两个工具都提供给模型来完成此任务。
我们将新工具添加到与之前相同的 DateTimeTools
类中。新工具将接受一个参数,即 ISO-8601 格式的时间。然后,该工具将向控制台打印一条消息,指示已为给定时间设置了闹钟。与之前一样,该工具被定义为一个使用 @Tool
注解的方法,我们还使用它来提供详细描述,以帮助模型理解何时以及如何使用该工具。
import java.time.LocalDateTime;
import java.time.format.DateTimeFormatter;
import org.springframework.ai.tool.annotation.Tool;
import org.springframework.context.i18n.LocaleContextHolder;
class DateTimeTools {
@Tool(description = "Get the current date and time in the user's timezone")
String getCurrentDateTime() {
return LocalDateTime.now().atZone(LocaleContextHolder.getTimeZone().toZoneId()).toString();
}
@Tool(description = "Set a user alarm for the given time, provided in ISO-8601 format")
void setAlarm(String time) {
LocalDateTime alarmTime = LocalDateTime.parse(time, DateTimeFormatter.ISO_DATE_TIME);
System.out.println("Alarm set for " + alarmTime);
}
}
接下来,让我们使这两个工具对模型可用。我们将使用 ChatClient
与模型交互。我们将通过 tools()
方法传递 DateTimeTools
的实例,从而向模型提供这两个工具。当我们要求设置一个从现在起 10 分钟后的闹钟时,模型首先需要知道当前日期和时间。然后,它将使用当前日期和时间计算闹钟时间。最后,它将使用闹钟工具设置闹钟。在内部,ChatClient
将处理来自模型的任何工具调用请求,并将任何工具调用执行结果发回给模型,以便模型生成最终响应。
ChatModel chatModel = ...
String response = ChatClient.create(chatModel)
.prompt("Can you set an alarm 10 minutes from now?")
.tools(new DateTimeTools())
.call()
.content();
System.out.println(response);
在应用日志中,您可以检查闹钟是否已在正确的时间设置。
概览
Spring AI 通过一组灵活的抽象支持工具调用,允许您以一致的方式定义、解析和执行工具。本节概述了 Spring AI 中工具调用的主要概念和组件。
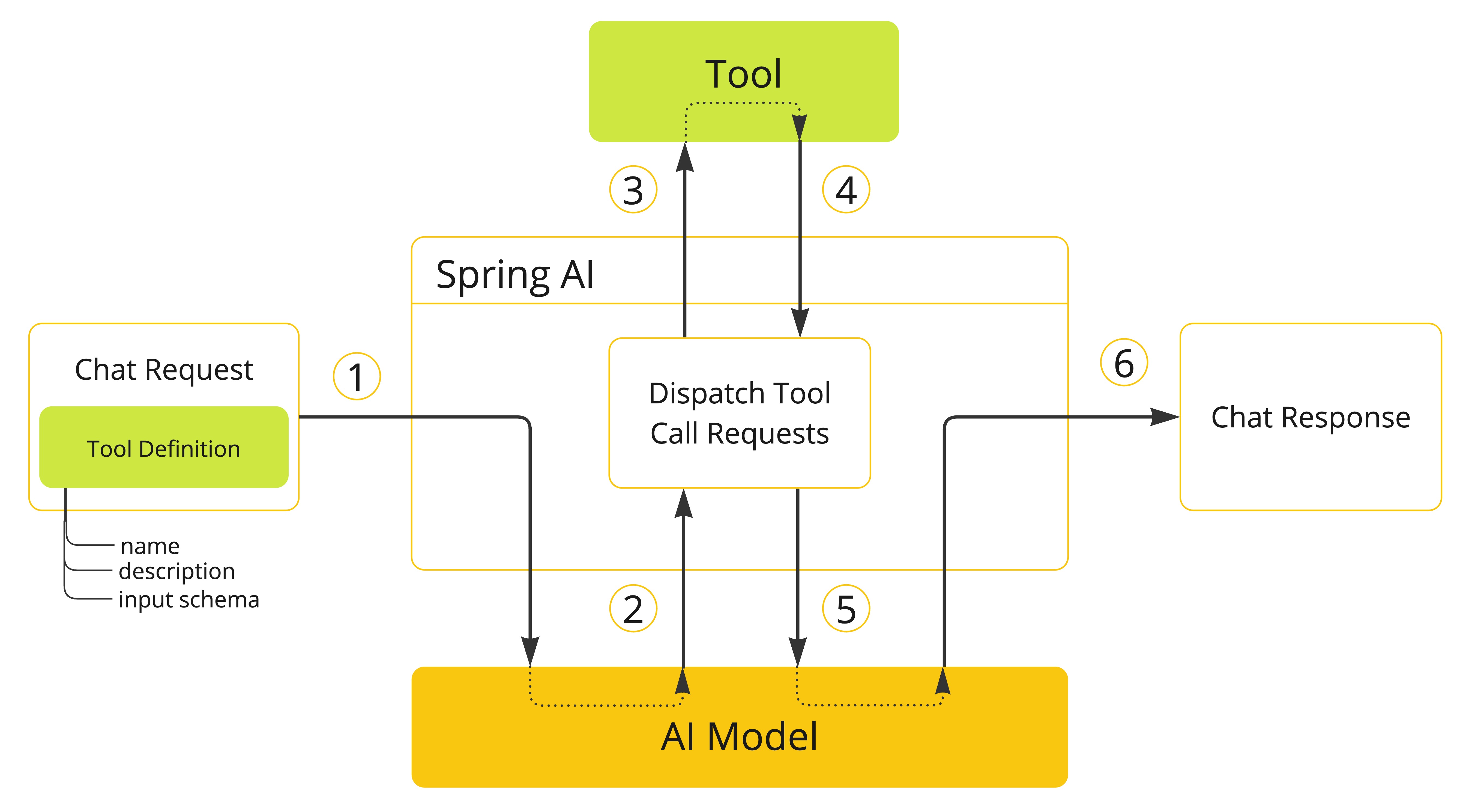
-
当我们想让工具对模型可用时,我们会将它的定义包含在聊天请求中。每个工具定义包括名称、描述和输入参数的模式。
-
当模型决定调用工具时,它会发送一个响应,其中包含工具名称以及根据定义模式建模的输入参数。
-
应用负责使用工具名称来识别并执行带有提供输入参数的工具。
-
工具调用的结果由应用处理。
-
应用将工具调用结果发送回模型。
-
模型使用工具调用结果作为附加上下文生成最终响应。
工具是工具调用的基本构建块,它们由 ToolCallback
接口建模。Spring AI 提供了内置支持,可以从方法和函数中指定 ToolCallback
,但您始终可以定义自己的 ToolCallback
实现以支持更多用例。
ChatModel
实现会透明地将工具调用请求分派给相应的 ToolCallback
实现,并将工具调用结果发送回模型,模型最终将生成最终响应。它们使用 ToolCallingManager
接口执行此操作,该接口负责管理工具执行生命周期。
ChatClient
和 ChatModel
都接受一个 ToolCallback
对象列表,以便将工具提供给模型以及最终将执行它们的 ToolCallingManager
。
除了直接传递 ToolCallback
对象外,您还可以传递一个工具名称列表,这些名称将使用 ToolCallbackResolver
接口进行动态解析。
以下部分将更详细地介绍所有这些概念和 API,包括如何自定义和扩展它们以支持更多用例。
方法作为工具
Spring AI 提供内置支持,可以通过两种方式从方法中指定工具(即 ToolCallback
):
-
声明式地,使用
@Tool
注解 -
编程式地,使用低级
MethodToolCallback
实现。
声明式规范:@Tool
您可以通过使用 @Tool
注解方法将其转换为工具。
class DateTimeTools {
@Tool(description = "Get the current date and time in the user's timezone")
String getCurrentDateTime() {
return LocalDateTime.now().atZone(LocaleContextHolder.getTimeZone().toZoneId()).toString();
}
}
@Tool
注解允许您提供关于工具的关键信息
-
name
:工具的名称。如果未提供,将使用方法名称。AI 模型在调用工具时使用此名称进行识别。因此,同一类中不允许存在名称相同的两个工具。对于特定聊天请求,该名称在模型可用的所有工具中必须是唯一的。 -
description
:工具的描述,模型可以使用此描述来理解何时以及如何调用该工具。如果未提供,将使用方法名称作为工具描述。但是,强烈建议提供详细描述,这对于模型理解工具目的及使用方法至关重要。未提供良好的描述可能导致模型在应该使用时未使用工具,或者使用错误。 -
returnDirect
:工具结果是直接返回给客户端,还是传回给模型。详情请参阅直接返回。 -
resultConverter
:用于将工具调用结果转换为要发送回 AI 模型的String
对象的ToolCallResultConverter
实现。详情请参阅结果转换。
方法可以是静态方法或实例方法,并且可以具有任何可见性(public、protected、package-private 或 private)。包含该方法的类可以是顶级类或嵌套类,并且也可以具有任何可见性(只要在您计划实例化它的地方可访问即可)。
只要包含方法的类是 Spring bean(例如 @Component ),Spring AI 就提供内置支持,对使用 @Tool 注解的方法进行 AOT 编译。否则,您需要向 GraalVM 编译器提供必要的配置。例如,通过使用 @RegisterReflection(memberCategories = MemberCategory.INVOKE_DECLARED_METHODS) 注解类。 |
您可以为方法定义任意数量的参数(包括无参数),参数类型可以是大多数类型(基本类型、POJO、枚举、列表、数组、Map 等等)。类似地,方法可以返回大多数类型,包括 void
。如果方法返回一个值,则返回类型必须是可序列化类型,因为结果将被序列化并发送回模型。
某些类型当前不支持。详情请参阅方法工具限制。 |
Spring AI 将自动为使用 @Tool
注解的方法的输入参数生成 JSON schema。schema 由模型用于理解如何调用工具并准备工具请求。@ToolParam
注解可用于提供关于输入参数的附加信息,例如描述或参数是必需还是可选。默认情况下,所有输入参数都被视为必需。
import java.time.LocalDateTime;
import java.time.format.DateTimeFormatter;
import org.springframework.ai.tool.annotation.Tool;
import org.springframework.ai.tool.annotation.ToolParam;
class DateTimeTools {
@Tool(description = "Set a user alarm for the given time")
void setAlarm(@ToolParam(description = "Time in ISO-8601 format") String time) {
LocalDateTime alarmTime = LocalDateTime.parse(time, DateTimeFormatter.ISO_DATE_TIME);
System.out.println("Alarm set for " + alarmTime);
}
}
@ToolParam
注解允许您提供关于工具参数的关键信息
-
description
:参数的描述,模型可以使用此描述更好地理解如何使用该参数。例如,参数应采用什么格式,允许什么值,等等。 -
required
:参数是必需的还是可选的。默认情况下,所有参数都被视为必需。
如果参数被标记为 @Nullable
,则它将被视为可选,除非使用 @ToolParam
注解明确标记为必需。
除了 @ToolParam
注解外,您还可以使用 Swagger 的 @Schema
注解或 Jackson 的 @JsonProperty
注解。详情请参阅JSON Schema。
向 ChatClient
添加工具
当使用声明式规范方法时,您可以在调用 ChatClient
时将工具类实例传递给 tools()
方法。此类工具仅对它们所添加到的特定聊天请求可用。
ChatClient.create(chatModel)
.prompt("What day is tomorrow?")
.tools(new DateTimeTools())
.call()
.content();
在底层,ChatClient
将从工具类实例中每个使用 @Tool
注解的方法生成一个 ToolCallback
,并将它们传递给模型。如果您更喜欢自己生成 ToolCallback
,可以使用 ToolCallbacks
工具类。
ToolCallback[] dateTimeTools = ToolCallbacks.from(new DateTimeTools());
向 ChatClient
添加默认工具
当使用声明式规范方法时,您可以通过将工具类实例传递给 defaultTools()
方法来向 ChatClient.Builder
添加默认工具。如果同时提供了默认工具和运行时工具,运行时工具将完全覆盖默认工具。
默认工具在由同一个 ChatClient.Builder 构建的所有 ChatClient 实例执行的所有聊天请求中共享。它们对于在不同聊天请求中常用的工具很有用,但如果使用不当,也可能存在危险,可能会在不应该可用的情况下使其可用。 |
ChatModel chatModel = ...
ChatClient chatClient = ChatClient.builder(chatModel)
.defaultTools(new DateTimeTools())
.build();
向 ChatModel
添加工具
当使用声明式规范方法时,您可以将工具类实例传递给您用于调用 ChatModel
的 ToolCallingChatOptions
的 toolCallbacks()
方法。此类工具仅对它们所添加到的特定聊天请求可用。
ChatModel chatModel = ...
ToolCallback[] dateTimeTools = ToolCallbacks.from(new DateTimeTools());
ChatOptions chatOptions = ToolCallingChatOptions.builder()
.toolCallbacks(dateTimeTools)
.build();
Prompt prompt = new Prompt("What day is tomorrow?", chatOptions);
chatModel.call(prompt);
向 ChatModel
添加默认工具
当使用声明式规范方法时,您可以在构建 ChatModel
时,通过将工具类实例传递给用于创建 ChatModel
的 ToolCallingChatOptions
实例的 toolCallbacks()
方法来添加默认工具。如果同时提供了默认工具和运行时工具,运行时工具将完全覆盖默认工具。
默认工具在该 ChatModel 实例执行的所有聊天请求中共享。它们对于在不同聊天请求中常用的工具很有用,但如果使用不当,也可能存在危险,可能会在不应该可用的情况下使其可用。 |
ToolCallback[] dateTimeTools = ToolCallbacks.from(new DateTimeTools());
ChatModel chatModel = OllamaChatModel.builder()
.ollamaApi(OllamaApi.builder().build())
.defaultOptions(ToolCallingChatOptions.builder()
.toolCallbacks(dateTimeTools)
.build())
.build();
编程式规范:MethodToolCallback
您可以通过编程式构建 MethodToolCallback
将方法转换为工具。
class DateTimeTools {
String getCurrentDateTime() {
return LocalDateTime.now().atZone(LocaleContextHolder.getTimeZone().toZoneId()).toString();
}
}
MethodToolCallback.Builder
允许您构建 MethodToolCallback
实例并提供关于工具的关键信息
-
toolDefinition
:定义工具名称、描述和输入 schema 的ToolDefinition
实例。您可以使用ToolDefinition.Builder
类构建它。必需。 -
toolMetadata
:定义附加设置(例如结果是否应直接返回给客户端以及要使用的结果转换器)的ToolMetadata
实例。您可以使用ToolMetadata.Builder
类构建它。 -
toolMethod
:表示工具方法的Method
实例。必需。 -
toolObject
:包含工具方法的对象实例。如果方法是静态方法,您可以省略此参数。 -
toolCallResultConverter
:用于将工具调用结果转换为要发送回 AI 模型的String
对象的ToolCallResultConverter
实例。如果未提供,将使用默认转换器 (DefaultToolCallResultConverter
)。
ToolDefinition.Builder
允许您构建 ToolDefinition
实例并定义工具名称、描述和输入 schema
-
name
:工具的名称。如果未提供,将使用方法名称。AI 模型在调用工具时使用此名称进行识别。因此,同一类中不允许存在名称相同的两个工具。对于特定聊天请求,该名称在模型可用的所有工具中必须是唯一的。 -
description
:工具的描述,模型可以使用此描述来理解何时以及如何调用该工具。如果未提供,将使用方法名称作为工具描述。但是,强烈建议提供详细描述,这对于模型理解工具目的及使用方法至关重要。未提供良好的描述可能导致模型在应该使用时未使用工具,或者使用错误。 -
inputSchema
:工具输入参数的 JSON schema。如果未提供,schema 将根据方法参数自动生成。您可以使用@ToolParam
注解提供有关输入参数的附加信息,例如描述或参数是必需还是可选。默认情况下,所有输入参数都被视为必需。详情请参阅JSON Schema。
ToolMetadata.Builder
允许您构建 ToolMetadata
实例并定义工具的附加设置
-
returnDirect
:工具结果是直接返回给客户端,还是传回给模型。详情请参阅直接返回。
Method method = ReflectionUtils.findMethod(DateTimeTools.class, "getCurrentDateTime");
ToolCallback toolCallback = MethodToolCallback.builder()
.toolDefinition(ToolDefinition.builder(method)
.description("Get the current date and time in the user's timezone")
.build())
.toolMethod(method)
.toolObject(new DateTimeTools())
.build();
方法可以是静态方法或实例方法,并且可以具有任何可见性(public、protected、package-private 或 private)。包含该方法的类可以是顶级类或嵌套类,并且也可以具有任何可见性(只要在您计划实例化它的地方可访问即可)。
只要包含方法的类是 Spring bean(例如 @Component ),Spring AI 就提供内置支持,对工具方法进行 AOT 编译。否则,您需要向 GraalVM 编译器提供必要的配置。例如,通过使用 @RegisterReflection(memberCategories = MemberCategory.INVOKE_DECLARED_METHODS) 注解类。 |
您可以为方法定义任意数量的参数(包括无参数),参数类型可以是大多数类型(基本类型、POJO、枚举、列表、数组、Map 等等)。类似地,方法可以返回大多数类型,包括 void
。如果方法返回一个值,则返回类型必须是可序列化类型,因为结果将被序列化并发送回模型。
某些类型当前不支持。详情请参阅方法工具限制。 |
如果方法是静态方法,您可以省略 toolObject()
方法,因为它不是必需的。
class DateTimeTools {
static String getCurrentDateTime() {
return LocalDateTime.now().atZone(LocaleContextHolder.getTimeZone().toZoneId()).toString();
}
}
Method method = ReflectionUtils.findMethod(DateTimeTools.class, "getCurrentDateTime");
ToolCallback toolCallback = MethodToolCallback.builder()
.toolDefinition(ToolDefinition.builder(method)
.description("Get the current date and time in the user's timezone")
.build())
.toolMethod(method)
.build();
Spring AI 将自动为方法的输入参数生成 JSON schema。schema 由模型用于理解如何调用工具并准备工具请求。@ToolParam
注解可用于提供关于输入参数的附加信息,例如描述或参数是必需还是可选。默认情况下,所有输入参数都被视为必需。
import java.time.LocalDateTime;
import java.time.format.DateTimeFormatter;
import org.springframework.ai.tool.annotation.ToolParam;
class DateTimeTools {
void setAlarm(@ToolParam(description = "Time in ISO-8601 format") String time) {
LocalDateTime alarmTime = LocalDateTime.parse(time, DateTimeFormatter.ISO_DATE_TIME);
System.out.println("Alarm set for " + alarmTime);
}
}
@ToolParam
注解允许您提供关于工具参数的关键信息
-
description
:参数的描述,模型可以使用此描述更好地理解如何使用该参数。例如,参数应采用什么格式,允许什么值,等等。 -
required
:参数是必需的还是可选的。默认情况下,所有参数都被视为必需。
如果参数被标记为 @Nullable
,则它将被视为可选,除非使用 @ToolParam
注解明确标记为必需。
除了 @ToolParam
注解外,您还可以使用 Swagger 的 @Schema
注解或 Jackson 的 @JsonProperty
注解。详情请参阅JSON Schema。
向 ChatClient
和 ChatModel
添加工具
当使用编程式规范方法时,您可以将 MethodToolCallback
实例传递给 ChatClient
的 tools()
方法。该工具仅对它所添加到的特定聊天请求可用。
ToolCallback toolCallback = ...
ChatClient.create(chatModel)
.prompt("What day is tomorrow?")
.tools(toolCallback)
.call()
.content();
向 ChatClient
添加默认工具
当使用编程式规范方法时,您可以通过将 MethodToolCallback
实例传递给 defaultTools()
方法来向 ChatClient.Builder
添加默认工具。如果同时提供了默认工具和运行时工具,运行时工具将完全覆盖默认工具。
默认工具在由同一个 ChatClient.Builder 构建的所有 ChatClient 实例执行的所有聊天请求中共享。它们对于在不同聊天请求中常用的工具很有用,但如果使用不当,也可能存在危险,可能会在不应该可用的情况下使其可用。 |
ChatModel chatModel = ...
ToolCallback toolCallback = ...
ChatClient chatClient = ChatClient.builder(chatModel)
.defaultTools(toolCallback)
.build();
向 ChatModel
添加工具
当使用编程式规范方法时,您可以将 MethodToolCallback
实例传递给您用于调用 ChatModel
的 ToolCallingChatOptions
的 toolCallbacks()
方法。该工具仅对它所添加到的特定聊天请求可用。
ChatModel chatModel = ...
ToolCallback toolCallback = ...
ChatOptions chatOptions = ToolCallingChatOptions.builder()
.toolCallbacks(toolCallback)
.build():
Prompt prompt = new Prompt("What day is tomorrow?", chatOptions);
chatModel.call(prompt);
向 ChatModel
添加默认工具
当使用编程式规范方法时,您可以在构建 ChatModel
时,通过将 MethodToolCallback
实例传递给用于创建 ChatModel
的 ToolCallingChatOptions
实例的 toolCallbacks()
方法来添加默认工具。如果同时提供了默认工具和运行时工具,运行时工具将完全覆盖默认工具。
默认工具在该 ChatModel 实例执行的所有聊天请求中共享。它们对于在不同聊天请求中常用的工具很有用,但如果使用不当,也可能存在危险,可能会在不应该可用的情况下使其可用。 |
ToolCallback toolCallback = ...
ChatModel chatModel = OllamaChatModel.builder()
.ollamaApi(OllamaApi.builder().build())
.defaultOptions(ToolCallingChatOptions.builder()
.toolCallbacks(toolCallback)
.build())
.build();
方法工具限制
以下类型当前不支持作为工具方法的参数或返回类型
-
Optional
-
异步类型(例如
CompletableFuture
,Future
) -
响应式类型(例如
Flow
,Mono
,Flux
) -
函数式类型(例如
Function
,Supplier
,Consumer
)。
函数式类型可以使用基于函数的工具规范方法来支持。详情请参阅函数作为工具。
函数作为工具
Spring AI 提供内置支持,可以从函数中指定工具,既可以通过编程式使用低级 FunctionToolCallback
实现,也可以动态地作为运行时解析的 @Bean
。
编程式规范:FunctionToolCallback
您可以通过编程式构建 FunctionToolCallback
将函数式类型(Function
、Supplier
、Consumer
或 BiFunction
)转换为工具。
public class WeatherService implements Function<WeatherRequest, WeatherResponse> {
public WeatherResponse apply(WeatherRequest request) {
return new WeatherResponse(30.0, Unit.C);
}
}
public enum Unit { C, F }
public record WeatherRequest(String location, Unit unit) {}
public record WeatherResponse(double temp, Unit unit) {}
FunctionToolCallback.Builder
允许您构建 FunctionToolCallback
实例并提供关于工具的关键信息
-
name
:工具的名称。AI 模型在调用工具时使用此名称进行识别。因此,在同一上下文中不允许存在名称相同的两个工具。对于特定聊天请求,该名称在模型可用的所有工具中必须是唯一的。必需。 -
toolFunction
:表示工具方法的函数式对象(Function
、Supplier
、Consumer
或BiFunction
)。必需。 -
description
:工具的描述,模型可以使用此描述来理解何时以及如何调用该工具。如果未提供,将使用方法名称作为工具描述。但是,强烈建议提供详细描述,这对于模型理解工具目的及使用方法至关重要。未提供良好的描述可能导致模型在应该使用时未使用工具,或者使用错误。 -
inputType
:函数输入的类型。必需。 -
inputSchema
:工具输入参数的 JSON schema。如果未提供,schema 将根据inputType
自动生成。您可以使用@ToolParam
注解提供有关输入参数的附加信息,例如描述或参数是必需还是可选。默认情况下,所有输入参数都被视为必需。详情请参阅JSON Schema。 -
toolMetadata
:定义附加设置(例如结果是否应直接返回给客户端以及要使用的结果转换器)的ToolMetadata
实例。您可以使用ToolMetadata.Builder
类构建它。 -
toolCallResultConverter
:用于将工具调用结果转换为要发送回 AI 模型的String
对象的ToolCallResultConverter
实例。如果未提供,将使用默认转换器 (DefaultToolCallResultConverter
)。
ToolMetadata.Builder
允许您构建 ToolMetadata
实例并定义工具的附加设置
-
returnDirect
:工具结果是直接返回给客户端,还是传回给模型。详情请参阅直接返回。
ToolCallback toolCallback = FunctionToolCallback
.builder("currentWeather", new WeatherService())
.description("Get the weather in location")
.inputType(WeatherRequest.class)
.build();
函数的输入和输出可以是 Void
或 POJO。输入和输出 POJO 必须是可序列化的,因为结果将被序列化并发送回模型。函数以及输入和输出类型必须是 public 的。
某些类型当前不支持。详情请参阅函数工具限制。 |
向 ChatClient
添加工具
当使用编程式规范方法时,您可以将 FunctionToolCallback
实例传递给 ChatClient
的 tools()
方法。该工具仅对它所添加到的特定聊天请求可用。
ToolCallback toolCallback = ...
ChatClient.create(chatModel)
.prompt("What's the weather like in Copenhagen?")
.tools(toolCallback)
.call()
.content();
向 ChatClient
添加默认工具
当使用编程式规范方法时,您可以通过将 FunctionToolCallback
实例传递给 defaultTools()
方法来向 ChatClient.Builder
添加默认工具。如果同时提供了默认工具和运行时工具,运行时工具将完全覆盖默认工具。
默认工具在由同一个 ChatClient.Builder 构建的所有 ChatClient 实例执行的所有聊天请求中共享。它们对于在不同聊天请求中常用的工具很有用,但如果使用不当,也可能存在危险,可能会在不应该可用的情况下使其可用。 |
ChatModel chatModel = ...
ToolCallback toolCallback = ...
ChatClient chatClient = ChatClient.builder(chatModel)
.defaultTools(toolCallback)
.build();
向 ChatModel
添加工具
当使用编程式规范方法时,您可以将 FunctionToolCallback
实例传递给 ToolCallingChatOptions
的 toolCallbacks()
方法。该工具仅对它所添加到的特定聊天请求可用。
ChatModel chatModel = ...
ToolCallback toolCallback = ...
ChatOptions chatOptions = ToolCallingChatOptions.builder()
.toolCallbacks(toolCallback)
.build():
Prompt prompt = new Prompt("What's the weather like in Copenhagen?", chatOptions);
chatModel.call(prompt);
向 ChatModel
添加默认工具
当使用编程式规范方法时,您可以在构建 ChatModel
时,通过将 FunctionToolCallback
实例传递给用于创建 ChatModel
的 ToolCallingChatOptions
实例的 toolCallbacks()
方法来添加默认工具。如果同时提供了默认工具和运行时工具,运行时工具将完全覆盖默认工具。
默认工具在该 ChatModel 实例执行的所有聊天请求中共享。它们对于在不同聊天请求中常用的工具很有用,但如果使用不当,也可能存在危险,可能会在不应该可用的情况下使其可用。 |
ToolCallback toolCallback = ...
ChatModel chatModel = OllamaChatModel.builder()
.ollamaApi(OllamaApi.builder().build())
.defaultOptions(ToolCallingChatOptions.builder()
.toolCallbacks(toolCallback)
.build())
.build();
动态规范:@Bean
除了以编程方式指定工具外,您还可以将工具定义为 Spring bean,并让 Spring AI 在运行时使用 ToolCallbackResolver
接口(通过 SpringBeanToolCallbackResolver
实现)动态解析它们。此选项使您可以使用任何 Function
、Supplier
、Consumer
或 BiFunction
bean 作为工具。bean 名称将用作工具名称,并且可以使用 Spring Framework 中的 @Description
注解来为工具提供描述,模型会使用此描述来理解何时以及如何调用该工具。如果您未提供描述,则方法名称将用作工具描述。但是,强烈建议提供详细的描述,因为这对于模型理解工具的用途以及如何使用它至关重要。未能提供良好的描述可能会导致模型在应该使用工具时未使用它,或者错误地使用它。
@Configuration(proxyBeanMethods = false)
class WeatherTools {
WeatherService weatherService = new WeatherService();
@Bean
@Description("Get the weather in location")
Function<WeatherRequest, WeatherResponse> currentWeather() {
return weatherService;
}
}
某些类型当前不支持。详情请参阅函数工具限制。 |
工具的输入参数的 JSON schema 将自动生成。您可以使用 @ToolParam
注解为输入参数提供额外信息,例如描述或参数是否为必需或可选。默认情况下,所有输入参数都被视为必需。有关更多详细信息,请参阅 JSON Schema。
record WeatherRequest(@ToolParam(description = "The name of a city or a country") String location, Unit unit) {}
这种工具规范方法存在一个缺点,即不保证类型安全,因为工具解析是在运行时完成的。为了弥补这一点,您可以使用 @Bean
注解明确指定工具名称,并将该值存储在一个常量中,以便您可以在聊天请求中使用它,而不是硬编码工具名称。
@Configuration(proxyBeanMethods = false)
class WeatherTools {
public static final String CURRENT_WEATHER_TOOL = "currentWeather";
@Bean(CURRENT_WEATHER_TOOL)
@Description("Get the weather in location")
Function<WeatherRequest, WeatherResponse> currentWeather() {
...
}
}
向 ChatClient
添加工具
使用动态规范方法时,您可以将工具名称(即函数 bean 名称)传递给 ChatClient
的 tools()
方法。该工具仅对添加到其中的特定聊天请求可用。
ChatClient.create(chatModel)
.prompt("What's the weather like in Copenhagen?")
.tools("currentWeather")
.call()
.content();
向 ChatClient
添加默认工具
使用动态规范方法时,您可以通过将工具名称传递给 defaultTools()
方法,向 ChatClient.Builder
添加默认工具。如果同时提供了默认工具和运行时工具,则运行时工具将完全覆盖默认工具。
默认工具在由同一个 ChatClient.Builder 构建的所有 ChatClient 实例执行的所有聊天请求中共享。它们对于在不同聊天请求中常用的工具很有用,但如果使用不当,也可能存在危险,可能会在不应该可用的情况下使其可用。 |
ChatModel chatModel = ...
ChatClient chatClient = ChatClient.builder(chatModel)
.defaultTools("currentWeather")
.build();
向 ChatModel
添加工具
使用动态规范方法时,您可以将工具名称传递给用于调用 ChatModel
的 ToolCallingChatOptions
的 toolNames()
方法。该工具仅对添加到其中的特定聊天请求可用。
ChatModel chatModel = ...
ChatOptions chatOptions = ToolCallingChatOptions.builder()
.toolNames("currentWeather")
.build():
Prompt prompt = new Prompt("What's the weather like in Copenhagen?", chatOptions);
chatModel.call(prompt);
向 ChatModel
添加默认工具
使用动态规范方法时,您可以在构建 ChatModel
时,通过将工具名称传递给用于创建 ChatModel
实例的 ToolCallingChatOptions
的 toolNames()
方法,向 ChatModel
添加默认工具。如果同时提供了默认工具和运行时工具,则运行时工具将完全覆盖默认工具。
默认工具在该 ChatModel 实例执行的所有聊天请求中共享。它们对于在不同聊天请求中常用的工具很有用,但如果使用不当,也可能存在危险,可能会在不应该可用的情况下使其可用。 |
ChatModel chatModel = OllamaChatModel.builder()
.ollamaApi(OllamaApi.builder().build())
.defaultOptions(ToolCallingChatOptions.builder()
.toolNames("currentWeather")
.build())
.build();
函数工具的限制
目前,以下类型不支持用作工具函数的输入或输出类型:
-
基本类型
-
Optional
-
集合类型(例如
List
、Map
、Array
、Set
) -
异步类型(例如
CompletableFuture
,Future
) -
响应式类型(例如
Flow
、Mono
、Flux
)。
基本类型和集合类型可以通过基于方法论的工具规范方法来支持。有关更多详细信息,请参阅 方法作为工具。
工具规范
在 Spring AI 中,工具通过 ToolCallback
接口建模。在前面的章节中,我们已经了解了如何使用 Spring AI 提供的内置支持(参见 方法作为工具 和 函数作为工具)从方法和函数定义工具。本节将深入探讨工具规范以及如何对其进行自定义和扩展以支持更多用例。
Tool Callback
ToolCallback
接口提供了一种定义可由 AI 模型调用的工具的方式,包括定义和执行逻辑。它是从头开始定义工具时需要实现的主要接口。例如,您可以从 MCP 客户端(使用 Model Context Protocol)或 ChatClient
定义 ToolCallback
(用于构建模块化代理应用程序)。
该接口提供以下方法:
public interface ToolCallback {
/**
* Definition used by the AI model to determine when and how to call the tool.
*/
ToolDefinition getToolDefinition();
/**
* Metadata providing additional information on how to handle the tool.
*/
ToolMetadata getToolMetadata();
/**
* Execute tool with the given input and return the result to send back to the AI model.
*/
String call(String toolInput);
/**
* Execute tool with the given input and context, and return the result to send back to the AI model.
*/
String call(String toolInput, ToolContext tooContext);
}
Spring AI 为工具方法(MethodToolCallback
)和工具函数(FunctionToolCallback
)提供了内置实现。
工具定义
ToolDefinition
接口为 AI 模型了解工具的可用性提供了所需信息,包括工具名称、描述和输入 schema。每个 ToolCallback
实现都必须提供一个 ToolDefinition
实例来定义工具。
该接口提供以下方法:
public interface ToolDefinition {
/**
* The tool name. Unique within the tool set provided to a model.
*/
String name();
/**
* The tool description, used by the AI model to determine what the tool does.
*/
String description();
/**
* The schema of the parameters used to call the tool.
*/
String inputSchema();
}
有关输入 schema 的更多详细信息,请参阅 JSON Schema。 |
ToolDefinition.Builder
使您能够使用默认实现(DefaultToolDefinition
)构建 ToolDefinition
实例。
ToolDefinition toolDefinition = ToolDefinition.builder()
.name("currentWeather")
.description("Get the weather in location")
.inputSchema("""
{
"type": "object",
"properties": {
"location": {
"type": "string"
},
"unit": {
"type": "string",
"enum": ["C", "F"]
}
},
"required": ["location", "unit"]
}
""")
.build();
方法工具定义
当从方法构建工具时,ToolDefinition
会自动为您生成。如果您更喜欢自己生成 ToolDefinition
,可以使用这个方便的构建器。
Method method = ReflectionUtils.findMethod(DateTimeTools.class, "getCurrentDateTime");
ToolDefinition toolDefinition = ToolDefinition.from(method);
从方法生成的 ToolDefinition
包括方法名称作为工具名称,方法名称作为工具描述,以及方法输入参数的 JSON schema。如果方法使用 @Tool
注解,则工具名称和描述(如果已设置)将取自该注解。
有关更多详细信息,请参阅 方法作为工具。 |
如果您宁愿显式提供部分或全部属性,可以使用 ToolDefinition.Builder
构建自定义的 ToolDefinition
实例。
Method method = ReflectionUtils.findMethod(DateTimeTools.class, "getCurrentDateTime");
ToolDefinition toolDefinition = ToolDefinition.builder(method)
.name("currentDateTime")
.description("Get the current date and time in the user's timezone")
.inputSchema(JsonSchemaGenerator.generateForMethodInput(method))
.build();
函数工具定义
当从函数构建工具时,ToolDefinition
会自动为您生成。当您使用 FunctionToolCallback.Builder
构建 FunctionToolCallback
实例时,您可以提供将用于生成 ToolDefinition
的工具名称、描述和输入 schema。有关更多详细信息,请参阅 函数作为工具。
JSON Schema
当向 AI 模型提供工具时,模型需要知道调用该工具所需的输入类型的 schema。Schema 用于理解如何调用工具并准备工具请求。Spring AI 通过 JsonSchemaGenerator
类提供内置支持,用于生成工具输入类型的 JSON Schema。该 schema 作为 ToolDefinition
的一部分提供。
有关 ToolDefinition 以及如何将输入 schema 传递给它的更多详细信息,请参阅 工具定义。 |
JsonSchemaGenerator
类在底层用于根据 方法作为工具 和 函数作为工具 中描述的任何策略,生成方法或函数输入参数的 JSON schema。JSON schema 生成逻辑支持一系列可用于方法和函数输入参数的注解,以自定义生成的 schema。
本节描述了在为工具输入参数生成 JSON schema 时可以自定义的两个主要选项:描述和必需状态。
描述
除了为工具本身提供描述外,您还可以为工具的输入参数提供描述。描述可用于提供有关输入参数的关键信息,例如参数应采用什么格式、允许什么值等。这有助于模型理解输入 schema 以及如何使用它。Spring AI 使用以下注解之一为输入参数提供内置支持:
-
Spring AI 的
@ToolParam(description = "…")
-
Jackson 的
@JsonClassDescription(description = "…")
-
Jackson 的
@JsonPropertyDescription(description = "…")
-
Swagger 的
@Schema(description = "…")
。
此方法适用于方法和函数,并且可以递归用于嵌套类型。
import java.time.LocalDateTime;
import java.time.format.DateTimeFormatter;
import org.springframework.ai.tool.annotation.Tool;
import org.springframework.ai.tool.annotation.ToolParam;
import org.springframework.context.i18n.LocaleContextHolder;
class DateTimeTools {
@Tool(description = "Set a user alarm for the given time")
void setAlarm(@ToolParam(description = "Time in ISO-8601 format") String time) {
LocalDateTime alarmTime = LocalDateTime.parse(time, DateTimeFormatter.ISO_DATE_TIME);
System.out.println("Alarm set for " + alarmTime);
}
}
必需/可选
默认情况下,每个输入参数都被视为必需,这会强制 AI 模型在调用工具时为其提供一个值。但是,您可以使用以下注解之一将输入参数设为可选,优先级顺序如下:
-
Spring AI 的
@ToolParam(required = false)
-
Jackson 的
@JsonProperty(required = false)
-
Swagger 的
@Schema(required = false)
-
Spring Framework 的
@Nullable
。
此方法适用于方法和函数,并且可以递归用于嵌套类型。
class CustomerTools {
@Tool(description = "Update customer information")
void updateCustomerInfo(Long id, String name, @ToolParam(required = false) String email) {
System.out.println("Updated info for customer with id: " + id);
}
}
定义正确的输入参数必需状态对于降低幻觉风险并确保模型在调用工具时提供正确的输入至关重要。在前面的示例中,email 参数是可选的,这意味着模型可以在不为其提供值的情况下调用该工具。如果该参数是必需的,则模型在调用工具时必须为其提供一个值。如果不存在值,模型可能会编造一个,从而导致幻觉。 |
结果转换
工具调用的结果使用 ToolCallResultConverter
进行序列化,然后发送回 AI 模型。ToolCallResultConverter
接口提供了一种将工具调用结果转换为 String
对象的方式。
该接口提供以下方法:
@FunctionalInterface
public interface ToolCallResultConverter {
/**
* Given an Object returned by a tool, convert it to a String compatible with the
* given class type.
*/
String convert(@Nullable Object result, @Nullable Type returnType);
}
结果必须是可序列化类型。默认情况下,结果使用 Jackson(DefaultToolCallResultConverter
)序列化为 JSON,但您可以通过提供自己的 ToolCallResultConverter
实现来自定义序列化过程。
Spring AI 在方法工具和函数工具中都依赖于 ToolCallResultConverter
。
方法工具调用结果转换
使用声明式方法从方法构建工具时,您可以通过设置 @Tool
注解的 resultConverter()
属性,为该工具提供自定义的 ToolCallResultConverter
。
class CustomerTools {
@Tool(description = "Retrieve customer information", resultConverter = CustomToolCallResultConverter.class)
Customer getCustomerInfo(Long id) {
return customerRepository.findById(id);
}
}
如果使用编程方式,您可以通过设置 MethodToolCallback.Builder
的 resultConverter()
属性,为该工具提供自定义的 ToolCallResultConverter
。
有关更多详细信息,请参阅 方法作为工具。
函数工具调用结果转换
使用编程方式从函数构建工具时,您可以通过设置 FunctionToolCallback.Builder
的 resultConverter()
属性,为该工具提供自定义的 ToolCallResultConverter
。
有关更多详细信息,请参阅 函数作为工具。
工具上下文
Spring AI 支持通过 ToolContext
API 将额外的上下文信息传递给工具。此功能使您能够提供额外的用户提供的数据,这些数据可以在工具执行过程中与 AI 模型传递的工具参数一起使用。
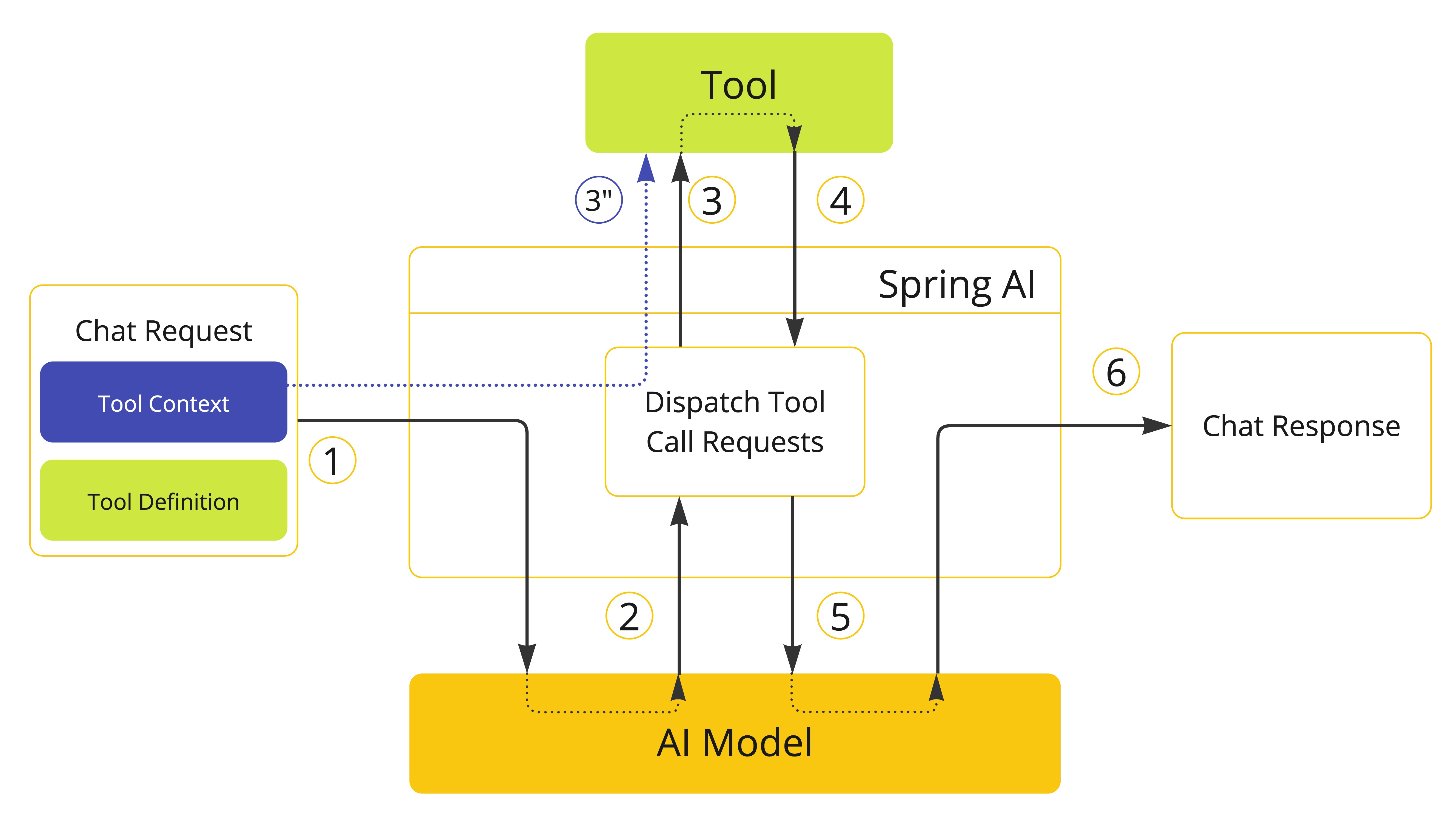
class CustomerTools {
@Tool(description = "Retrieve customer information")
Customer getCustomerInfo(Long id, ToolContext toolContext) {
return customerRepository.findById(id, toolContext.get("tenantId"));
}
}
在调用 ChatClient
时,ToolContext
会填充用户提供的数据。
ChatModel chatModel = ...
String response = ChatClient.create(chatModel)
.prompt("Tell me more about the customer with ID 42")
.tools(new CustomerTools())
.toolContext(Map.of("tenantId", "acme"))
.call()
.content();
System.out.println(response);
ToolContext 中提供的任何数据都不会发送给 AI 模型。 |
同样,在直接调用 ChatModel
时,您可以定义工具上下文数据。
ChatModel chatModel = ...
ToolCallback[] customerTools = ToolCallbacks.from(new CustomerTools());
ChatOptions chatOptions = ToolCallingChatOptions.builder()
.toolCallbacks(customerTools)
.toolContext(Map.of("tenantId", "acme"))
.build();
Prompt prompt = new Prompt("Tell me more about the customer with ID 42", chatOptions);
chatModel.call(prompt);
如果在默认选项和运行时选项中都设置了 toolContext
选项,则生成的 ToolContext
将是两者的合并,其中运行时选项优先于默认选项。
直接返回
默认情况下,工具调用的结果作为响应发送回模型。然后,模型可以使用该结果继续对话。
有些情况下,您可能希望直接将结果返回给调用者,而不是将其发送回模型。例如,如果您构建了一个依赖 RAG 工具的代理,您可能希望将结果直接返回给调用者,而不是将其发送回模型进行不必要的后处理。或者,您可能有一些工具应该结束代理的推理循环。
每个 ToolCallback
实现都可以定义工具调用的结果是直接返回给调用者还是发送回模型。默认情况下,结果会发送回模型。但您可以针对每个工具更改此行为。
负责管理工具执行生命周期的 ToolCallingManager
负责处理与工具关联的 returnDirect
属性。如果此属性设置为 true
,则工具调用的结果将直接返回给调用者。否则,结果会发送回模型。
如果同时请求多个工具调用,则所有工具的 returnDirect 属性都必须设置为 true ,才能将结果直接返回给调用者。否则,结果将发送回模型。 |
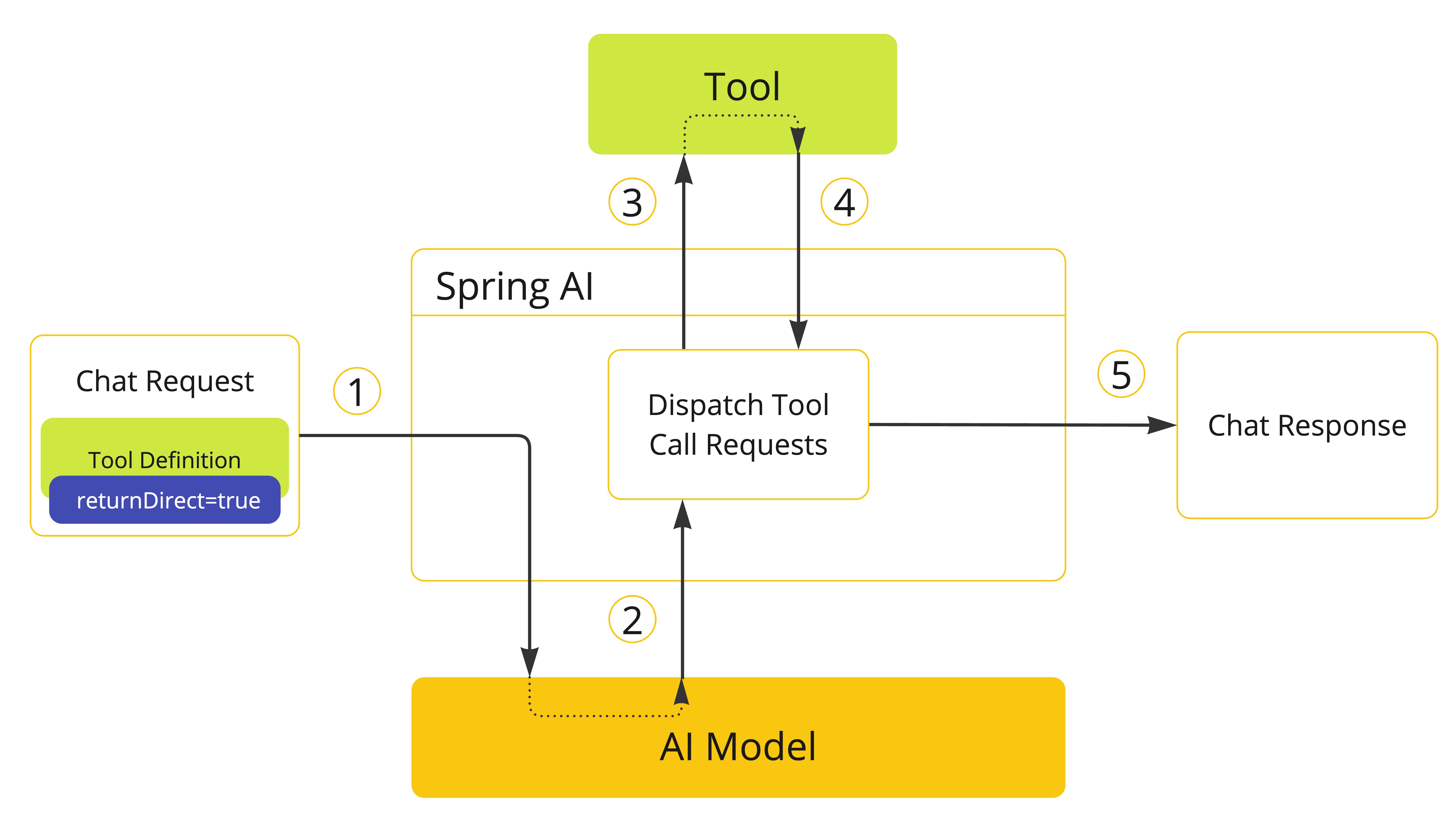
-
当我们想让模型可以使用某个工具时,我们会将该工具的定义包含在聊天请求中。如果希望工具执行的结果直接返回给调用者,我们将
returnDirect
属性设置为true
。 -
当模型决定调用工具时,它会发送一个响应,其中包含工具名称以及根据定义模式建模的输入参数。
-
应用负责使用工具名称来识别并执行带有提供输入参数的工具。
-
工具调用的结果由应用处理。
-
应用程序直接将工具调用结果发送给调用者,而不是将其发送回模型。
方法直接返回
使用声明式方法从方法构建工具时,您可以通过将 @Tool
注解的 returnDirect
属性设置为 true
,将工具标记为直接将结果返回给调用者。
class CustomerTools {
@Tool(description = "Retrieve customer information", returnDirect = true)
Customer getCustomerInfo(Long id) {
return customerRepository.findById(id);
}
}
如果使用编程方式,您可以通过 ToolMetadata
接口设置 returnDirect
属性,并将其传递给 MethodToolCallback.Builder
。
ToolMetadata toolMetadata = ToolMetadata.builder()
.returnDirect(true)
.build();
有关更多详细信息,请参阅 方法作为工具。
函数直接返回
使用编程方式从函数构建工具时,您可以通过 ToolMetadata
接口设置 returnDirect
属性,并将其传递给 FunctionToolCallback.Builder
。
ToolMetadata toolMetadata = ToolMetadata.builder()
.returnDirect(true)
.build();
有关更多详细信息,请参阅 函数作为工具。
工具执行
工具执行是指使用提供的输入参数调用工具并返回结果的过程。工具执行由负责管理工具执行生命周期的 ToolCallingManager
接口处理。
public interface ToolCallingManager {
/**
* Resolve the tool definitions from the model's tool calling options.
*/
List<ToolDefinition> resolveToolDefinitions(ToolCallingChatOptions chatOptions);
/**
* Execute the tool calls requested by the model.
*/
ToolExecutionResult executeToolCalls(Prompt prompt, ChatResponse chatResponse);
}
如果您正在使用任何 Spring AI Spring Boot Starter,则 DefaultToolCallingManager
是 ToolCallingManager
接口的自动配置实现。您可以通过提供自己的 ToolCallingManager
bean 来自定义工具执行行为。
@Bean
ToolCallingManager toolCallingManager() {
return ToolCallingManager.builder().build();
}
默认情况下,Spring AI 在每个 ChatModel
实现内部透明地为您管理工具执行生命周期。但您可以选择退出此行为并自行控制工具执行。本节描述了这两种场景。
框架控制的工具执行
使用默认行为时,Spring AI 会自动拦截来自模型的任何工具调用请求,调用工具并将结果返回给模型。所有这一切都由每个 ChatModel
实现使用 ToolCallingManager
透明地为您完成。
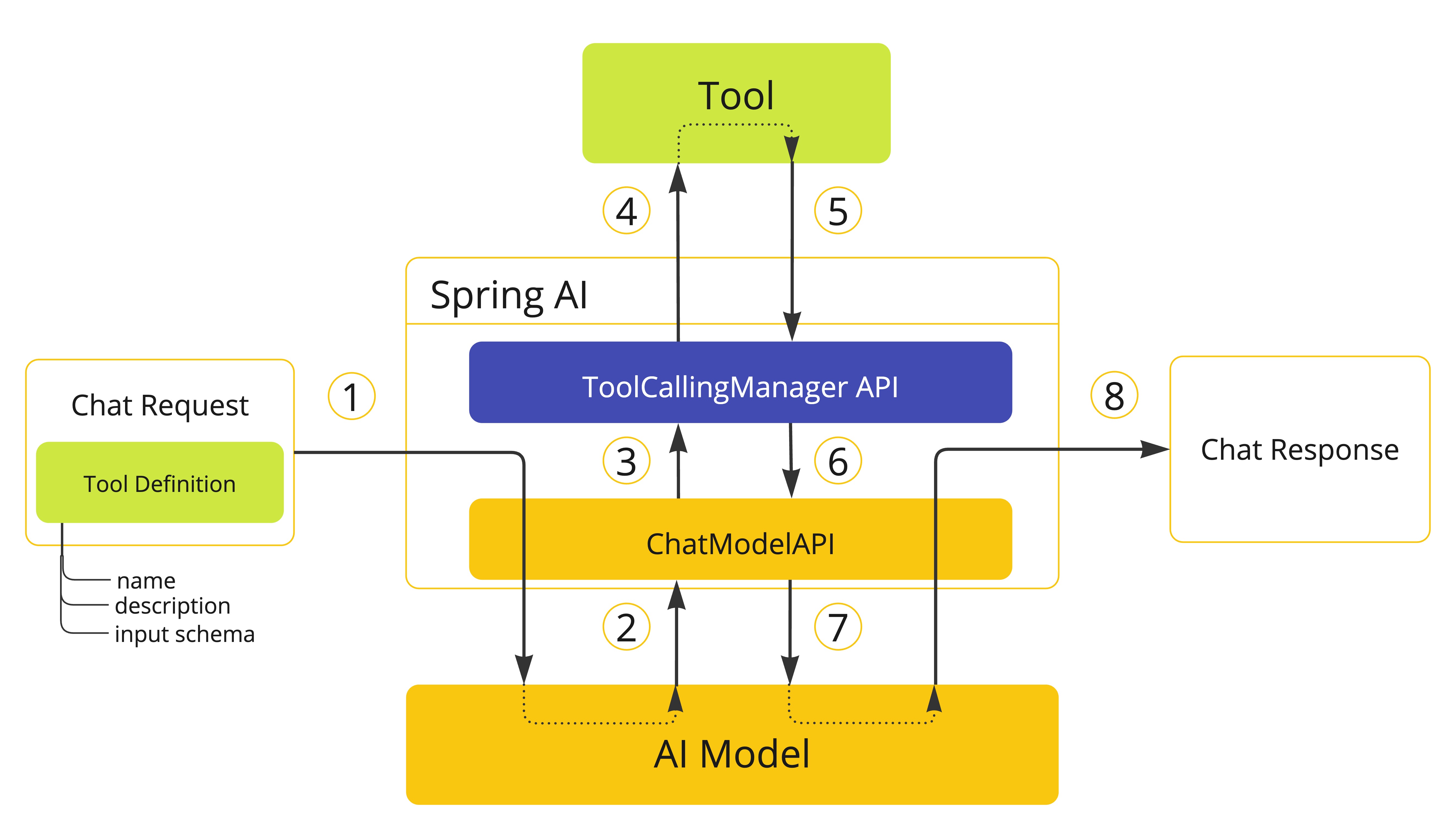
-
当我们想让模型可以使用某个工具时,我们会将该工具的定义包含在聊天请求(
Prompt
)中,并调用将请求发送到 AI 模型的ChatModel
API。 -
当模型决定调用某个工具时,它会发送一个响应(
ChatResponse
),其中包含工具名称和根据已定义 schema 建模的输入参数。 -
ChatModel
将工具调用请求发送到ToolCallingManager
API。 -
ToolCallingManager
负责识别要调用的工具,并使用提供的输入参数执行它。 -
工具调用的结果返回给
ToolCallingManager
。 -
ToolCallingManager
将工具执行结果返回给ChatModel
。 -
ChatModel
将工具执行结果发送回 AI 模型(ToolResponseMessage
)。 -
AI 模型使用工具调用结果作为额外上下文生成最终响应,并通过
ChatClient
将其发送回调用者(ChatResponse
)。
目前,与模型之间关于工具执行的内部消息不会暴露给用户。如果您需要访问这些消息,应使用用户控制的工具执行方法。 |
决定工具调用是否符合执行条件的逻辑由 ToolExecutionEligibilityPredicate
接口处理。默认情况下,工具执行资格通过检查 ToolCallingChatOptions
的 internalToolExecutionEnabled
属性是否设置为 true
(默认值),以及 ChatResponse
是否包含任何工具调用来确定。
public class DefaultToolExecutionEligibilityPredicate implements ToolExecutionEligibilityPredicate {
@Override
public boolean test(ChatOptions promptOptions, ChatResponse chatResponse) {
return ToolCallingChatOptions.isInternalToolExecutionEnabled(promptOptions) && chatResponse != null
&& chatResponse.hasToolCalls();
}
}
在创建 ChatModel
bean 时,您可以提供自定义的 ToolExecutionEligibilityPredicate
实现。
用户控制的工具执行
有些情况下,您可能希望自行控制工具执行生命周期。您可以通过将 ToolCallingChatOptions
的 internalToolExecutionEnabled
属性设置为 false
来实现此目的。
使用此选项调用 ChatModel
时,工具执行将被委托给调用者,使您可以完全控制工具执行生命周期。您有责任检查 ChatResponse
中的工具调用,并使用 ToolCallingManager
执行它们。
以下示例演示了用户控制的工具执行方法的最小实现:
ChatModel chatModel = ...
ToolCallingManager toolCallingManager = ToolCallingManager.builder().build();
ChatOptions chatOptions = ToolCallingChatOptions.builder()
.toolCallbacks(new CustomerTools())
.internalToolExecutionEnabled(false)
.build();
Prompt prompt = new Prompt("Tell me more about the customer with ID 42", chatOptions);
ChatResponse chatResponse = chatModel.call(prompt);
while (chatResponse.hasToolCalls()) {
ToolExecutionResult toolExecutionResult = toolCallingManager.executeToolCalls(prompt, chatResponse);
prompt = new Prompt(toolExecutionResult.conversationHistory(), chatOptions);
chatResponse = chatModel.call(prompt);
}
System.out.println(chatResponse.getResult().getOutput().getText());
选择用户控制的工具执行方法时,我们建议使用 ToolCallingManager 来管理工具调用操作。这样,您可以受益于 Spring AI 提供的工具执行内置支持。但是,您也可以实现自己的工具执行逻辑。 |
接下来的示例展示了用户控制的工具执行方法与 ChatMemory
API 使用相结合的最小实现:
ToolCallingManager toolCallingManager = DefaultToolCallingManager.builder().build();
ChatMemory chatMemory = MessageWindowChatMemory.builder().build();
String conversationId = UUID.randomUUID().toString();
ChatOptions chatOptions = ToolCallingChatOptions.builder()
.toolCallbacks(ToolCallbacks.from(new MathTools()))
.internalToolExecutionEnabled(false)
.build();
Prompt prompt = new Prompt(
List.of(new SystemMessage("You are a helpful assistant."), new UserMessage("What is 6 * 8?")),
chatOptions);
chatMemory.add(conversationId, prompt.getInstructions());
Prompt promptWithMemory = new Prompt(chatMemory.get(conversationId), chatOptions);
ChatResponse chatResponse = chatModel.call(promptWithMemory);
chatMemory.add(conversationId, chatResponse.getResult().getOutput());
while (chatResponse.hasToolCalls()) {
ToolExecutionResult toolExecutionResult = toolCallingManager.executeToolCalls(promptWithMemory,
chatResponse);
chatMemory.add(conversationId, toolExecutionResult.conversationHistory()
.get(toolExecutionResult.conversationHistory().size() - 1));
promptWithMemory = new Prompt(chatMemory.get(conversationId), chatOptions);
chatResponse = chatModel.call(promptWithMemory);
chatMemory.add(conversationId, chatResponse.getResult().getOutput());
}
UserMessage newUserMessage = new UserMessage("What did I ask you earlier?");
chatMemory.add(conversationId, newUserMessage);
ChatResponse newResponse = chatModel.call(new Prompt(chatMemory.get(conversationId)));
异常处理
当工具调用失败时,异常将作为 ToolExecutionException
传播,可以捕获该异常来处理错误。ToolExecutionExceptionProcessor
可用于处理 ToolExecutionException
,它有两种结果:生成一个错误消息发送回 AI 模型,或者抛出一个异常由调用者处理。
@FunctionalInterface
public interface ToolExecutionExceptionProcessor {
/**
* Convert an exception thrown by a tool to a String that can be sent back to the AI
* model or throw an exception to be handled by the caller.
*/
String process(ToolExecutionException exception);
}
如果您正在使用任何 Spring AI Spring Boot Starter,则 DefaultToolExecutionExceptionProcessor
是 ToolExecutionExceptionProcessor
接口的自动配置实现。默认情况下,错误消息会发送回模型。DefaultToolExecutionExceptionProcessor
构造函数允许您将 alwaysThrow
属性设置为 true
或 false
。如果设置为 true
,则会抛出异常而不是将错误消息发送回模型。
@Bean
ToolExecutionExceptionProcessor toolExecutionExceptionProcessor() {
return new DefaultToolExecutionExceptionProcessor(true);
}
如果您定义了自己的 ToolCallback 实现,请确保在 call() 方法的工具执行逻辑中发生错误时抛出 ToolExecutionException 。 |
默认的 ToolCallingManager
(DefaultToolCallingManager
)内部使用 ToolExecutionExceptionProcessor
来处理工具执行期间的异常。有关工具执行生命周期的更多详细信息,请参阅 工具执行。
工具解析
但是,Spring AI 还支持使用 ToolCallbackResolver
接口在运行时动态解析工具。
public interface ToolCallbackResolver {
/**
* Resolve the {@link ToolCallback} for the given tool name.
*/
@Nullable
ToolCallback resolve(String toolName);
}
使用这种方法时:
-
在客户端,您向
ChatClient
或ChatModel
提供工具名称,而不是ToolCallback
(s)。 -
在服务端,
ToolCallbackResolver
实现负责将工具名称解析为相应的ToolCallback
实例。
默认情况下,Spring AI 依赖于 DelegatingToolCallbackResolver
,它将工具解析委托给 ToolCallbackResolver
实例列表:
-
SpringBeanToolCallbackResolver
从类型为Function
、Supplier
、Consumer
或BiFunction
的 Spring bean 中解析工具。有关更多详细信息,请参阅 动态规范:@Bean
。 -
StaticToolCallbackResolver
从ToolCallback
实例的静态列表中解析工具。使用 Spring Boot 自动配置时,此解析器会自动配置应用程序上下文中定义的所有ToolCallback
类型的 bean。
如果您依赖于 Spring Boot 自动配置,您可以通过提供自定义的 ToolCallbackResolver
bean 来自定义解析逻辑。
@Bean
ToolCallbackResolver toolCallbackResolver(List<FunctionCallback> toolCallbacks) {
StaticToolCallbackResolver staticToolCallbackResolver = new StaticToolCallbackResolver(toolCallbacks);
return new DelegatingToolCallbackResolver(List.of(staticToolCallbackResolver));
}