Cohere 嵌入
提供 Bedrock Cohere 嵌入模型。将生成式 AI 功能集成到提升业务成果的关键应用程序和工作流程中。
有关如何使用 AWS 托管模型的详细信息,请参阅 AWS Bedrock Cohere 模型页面 和 Amazon Bedrock 用户指南。
先决条件
有关设置 API 访问权限,请参阅 Amazon Bedrock 的 Spring AI 文档。
自动配置
将 spring-ai-bedrock-ai-spring-boot-starter
依赖项添加到项目的 Maven pom.xml
文件
<dependency>
<groupId>org.springframework.ai</groupId>
<artifactId>spring-ai-bedrock-ai-spring-boot-starter</artifactId>
</dependency>
或到您的 Gradle build.gradle
构建文件。
dependencies {
implementation 'org.springframework.ai:spring-ai-bedrock-ai-spring-boot-starter'
}
有关如何将 Spring AI BOM 添加到您的构建文件,请参阅 依赖项管理 部分。 |
启用 Cohere 嵌入支持
默认情况下,Cohere 模型处于禁用状态。要启用它,请将 spring.ai.bedrock.cohere.embedding.enabled
属性设置为 true
。导出环境变量是设置此配置属性的一种方法。
export SPRING_AI_BEDROCK_COHERE_EMBEDDING_ENABLED=true
嵌入属性
前缀 spring.ai.bedrock.aws
是配置与 AWS Bedrock 连接的属性前缀。
属性 | 描述 | 默认值 |
---|---|---|
spring.ai.bedrock.aws.region |
要使用的 AWS 区域。 |
us-east-1 |
spring.ai.bedrock.aws.access-key |
AWS 访问密钥。 |
- |
spring.ai.bedrock.aws.secret-key |
AWS 密钥。 |
- |
前缀 spring.ai.bedrock.cohere.embedding
(在 BedrockCohereEmbeddingProperties
中定义)是配置 Cohere 的嵌入模型实现的属性前缀。
属性 |
描述 |
默认值 |
spring.ai.bedrock.cohere.embedding.enabled |
启用或禁用对 Cohere 的支持 |
false |
spring.ai.bedrock.cohere.embedding.model |
要使用的模型 ID。有关支持的模型,请参阅 CohereEmbeddingModel。 |
cohere.embed-multilingual-v3 |
spring.ai.bedrock.cohere.embedding.options.input-type |
在前面添加特殊标记以区分每种类型。您不应混合使用不同类型,除非在混合用于搜索和检索的类型时。在这种情况下,使用 search_document 类型嵌入您的语料库,并使用 search_query 类型嵌入查询。 |
SEARCH_DOCUMENT |
spring.ai.bedrock.cohere.embedding.options.truncate |
指定 API 如何处理比最大标记长度更长的输入。如果指定 LEFT 或 RIGHT,则模型会丢弃输入,直到剩余输入恰好是模型的最大输入标记长度。 |
NONE |
查看 CohereEmbeddingModel 以获取其他模型 ID。支持的值为:cohere.embed-multilingual-v3
和 cohere.embed-english-v3
。模型 ID 值也可以在 AWS Bedrock 基础模型 ID 文档 中找到。
所有以 spring.ai.bedrock.cohere.embedding.options 为前缀的属性都可以在运行时通过向 EmbeddingRequest 调用添加特定于请求的 运行时选项 来覆盖。 |
运行时选项
BedrockCohereEmbeddingOptions.java 提供模型配置,例如 input-type
或 truncate
。
在启动时,可以使用 BedrockCohereEmbeddingModel(api, options)
构造函数或 spring.ai.bedrock.cohere.embedding.options.*
属性配置默认选项。
在运行时,您可以通过向 EmbeddingRequest
调用添加新的、特定于请求的选项来覆盖默认选项。例如,要覆盖特定请求的默认输入类型
EmbeddingResponse embeddingResponse = embeddingModel.call(
new EmbeddingRequest(List.of("Hello World", "World is big and salvation is near"),
BedrockCohereEmbeddingOptions.builder()
.withInputType(InputType.SEARCH_DOCUMENT)
.build()));
示例控制器
创建一个新的 Spring Boot 项目,并将 spring-ai-bedrock-ai-spring-boot-starter
添加到您的 pom(或 gradle)依赖项中。
在src/main/resources
目录下添加一个application.properties
文件,以启用和配置Cohere Embedding模型。
spring.ai.bedrock.aws.region=eu-central-1
spring.ai.bedrock.aws.access-key=${AWS_ACCESS_KEY_ID}
spring.ai.bedrock.aws.secret-key=${AWS_SECRET_ACCESS_KEY}
spring.ai.bedrock.cohere.embedding.enabled=true
spring.ai.bedrock.cohere.embedding.options.input-type=search-document
将regions 、access-key 和secret-key 替换为您的AWS凭证。 |
这将创建一个BedrockCohereEmbeddingModel
实现,您可以将其注入到您的类中。这是一个使用聊天模型进行文本生成的简单@Controller
类的示例。
@RestController
public class EmbeddingController {
private final EmbeddingModel embeddingModel;
@Autowired
public EmbeddingController(EmbeddingModel embeddingModel) {
this.embeddingModel = embeddingModel;
}
@GetMapping("/ai/embedding")
public Map embed(@RequestParam(value = "message", defaultValue = "Tell me a joke") String message) {
EmbeddingResponse embeddingResponse = this.embeddingModel.embedForResponse(List.of(message));
return Map.of("embedding", embeddingResponse);
}
}
手动配置
The BedrockCohereEmbeddingModel实现了EmbeddingModel
接口,并使用低级CohereEmbeddingBedrockApi客户端连接到Bedrock Cohere服务。
将spring-ai-bedrock
依赖项添加到您项目的Maven pom.xml
文件中。
<dependency>
<groupId>org.springframework.ai</groupId>
<artifactId>spring-ai-bedrock</artifactId>
</dependency>
或到您的 Gradle build.gradle
构建文件。
dependencies {
implementation 'org.springframework.ai:spring-ai-bedrock'
}
有关如何将 Spring AI BOM 添加到您的构建文件,请参阅 依赖项管理 部分。 |
接下来,创建一个BedrockCohereEmbeddingModel并将其用于文本嵌入。
var cohereEmbeddingApi =new CohereEmbeddingBedrockApi(
CohereEmbeddingModel.COHERE_EMBED_MULTILINGUAL_V1.id(),
EnvironmentVariableCredentialsProvider.create(), Region.US_EAST_1.id(), new ObjectMapper());
var embeddingModel = new BedrockCohereEmbeddingModel(this.cohereEmbeddingApi);
EmbeddingResponse embeddingResponse = this.embeddingModel
.embedForResponse(List.of("Hello World", "World is big and salvation is near"));
低级CohereEmbeddingBedrockApi客户端
The CohereEmbeddingBedrockApi提供了一个基于AWS Bedrock Cohere Command模型的轻量级Java客户端。
下面的类图说明了CohereEmbeddingBedrockApi接口和构建块。
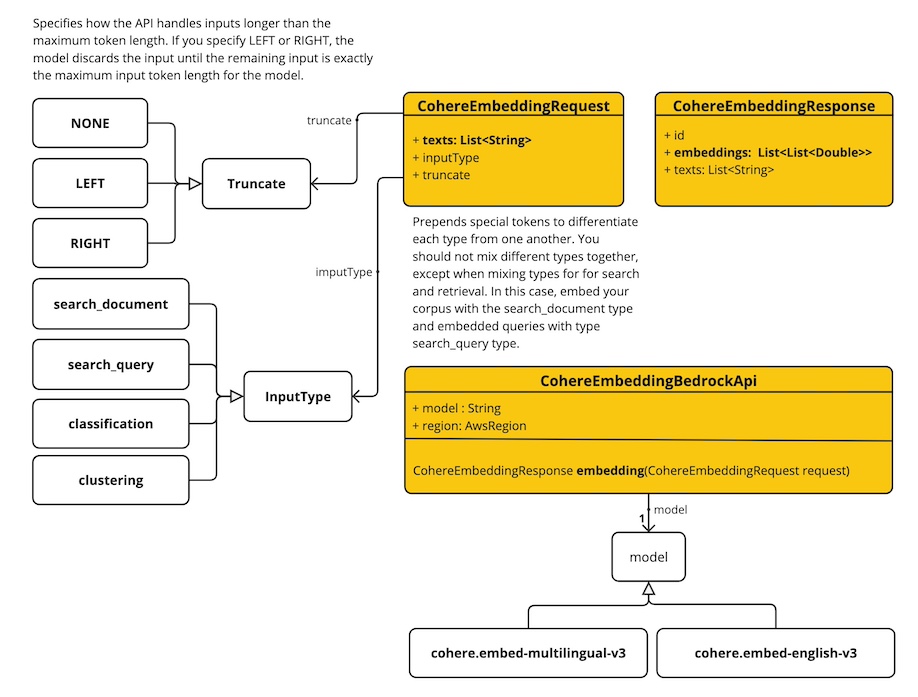
CohereEmbeddingBedrockApi支持cohere.embed-english-v3
和cohere.embed-multilingual-v3
模型进行单一和批量嵌入计算。
以下是一个简单的代码片段,演示如何以编程方式使用该API。
CohereEmbeddingBedrockApi api = new CohereEmbeddingBedrockApi(
CohereEmbeddingModel.COHERE_EMBED_MULTILINGUAL_V1.id(),
EnvironmentVariableCredentialsProvider.create(),
Region.US_EAST_1.id(), new ObjectMapper());
CohereEmbeddingRequest request = new CohereEmbeddingRequest(
List.of("I like to eat apples", "I like to eat oranges"),
CohereEmbeddingRequest.InputType.search_document,
CohereEmbeddingRequest.Truncate.NONE);
CohereEmbeddingResponse response = this.api.embedding(this.request);